My start with React and its rich ecosystem
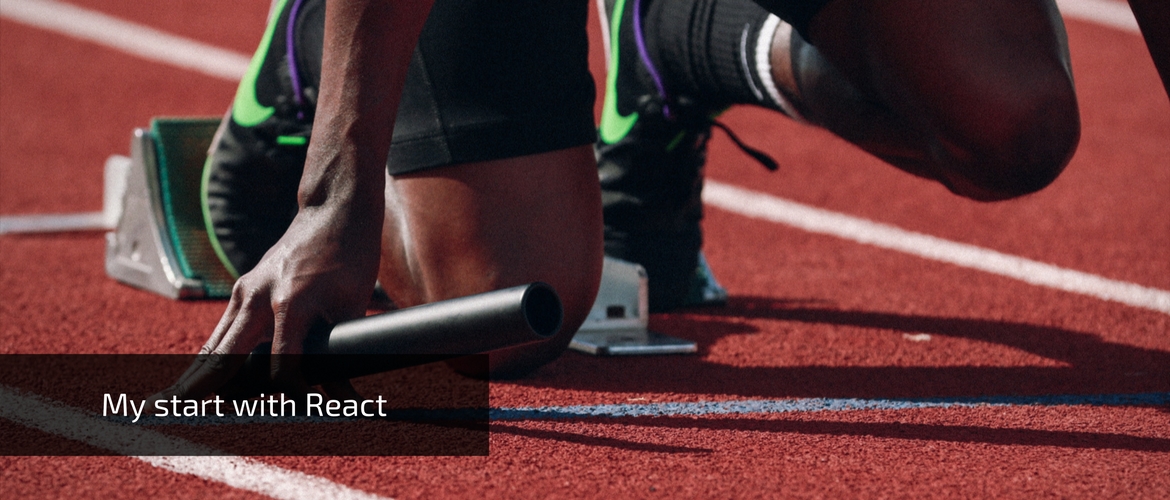
I remember how I was excited discovering how much frontend frameworks like AngularJS could make my work easier. Even though I was a strong backend-oriented programmer I decided to learn the concept of Angular. I dug into it at ease and I started using this tool in my daily work.
Since I changed my job I lost the opportunity to use frontend technologies regularly. However, I’m still fascinated about this part of software development and I follow trends and latest changes. Therefore AngularJS 1.x was officially abandoned I started looking for something new and fresh.
I didn’t look for a long time. When I first saw React library, I fall in love with it. I quickly caught the concept behind this tool, thanks to ubiquitous tutorials and examples. I read a lot about the flux pattern, redux, components but I didn’t chance to use them in something more ambitious than <HelloWorld/>
component. Till now.
Project conception
Because I still study and I have some projects to do, I decided to smuggle some new stuff this way. This is a really nice opportunity to try something new because of at least two reasons:
- You have a specified goal of project.
- Your project must work.
Simple, but enough. These rules prevent the further work from being abandonment in the half of project.
My project is a simple online password manager with client-side encryption. This sounds like a good playground for a React application. It also needs some server-side work but first I want to focus on the front-end.
I started working with React
When I first tried to work with React I was frightened how much work it needs to set up a simple environment. Some time ago I tried it once again using an official tool create-react-app
. A fresh React project was ready for several seconds. That’s impressive.
Bootstrap comes in
Because of time limitation, I was looking for some existing component libraries dedicated to the UI development. I didn’t want to reinvent the wheel. After a small research, I choose the reactstrap
library which wraps Bootstrap components into React ones. Great.
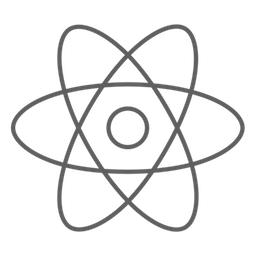
I also started developing my custom components. This is one example:
class PasswordField extends React.Component {
constructor() {
super();
this.state = {hidden: true};
}
toggleVisibility() {
this.setState((prevState) => ({hidden: !prevState.hidden}));
}
render() {
return (
<div className="PasswordField">
<InputGroup>
<InputGroupAddon>
<FaEye className="PasswordField-Icon" onClick={this.toggleVisibility.bind(this)}/>
</InputGroupAddon>
<Input value={this.state.hidden ? '∗∗∗∗∗∗∗∗∗∗∗∗∗∗∗∗∗∗∗∗∗' : this.props.password} readOnly={true}/>
</InputGroup>
</div>
);
}
}
Needs for routing
After prototyping one screen I realized that could be nice to have a router to add some interactions to my application. Another research led me to the react-router library. Some examples from documentation gave me a good playground.
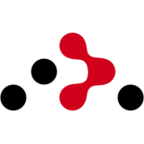
I’m a little bit scary reading how these things change over time. According to official documentation, react-router have been completely rewritten. It makes a migration to the newest version difficult.
State management
Subsequently, I added redux to my application. I learned about the concept behind this in past mostly from articles and video courses. Dan Abramov created a brief video introduction to redux – it’s a great starter. Now I check there in the action and I feel that without the good background it would be hard to use it ad hoc.
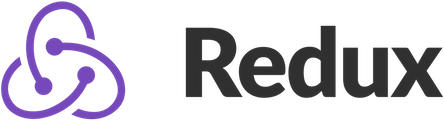
In React, I have to describe all changes explicitly. Even when it comes to binding input into the component’s state. Switching from two-way binding to this is awkward but I know problems with bindings within AngularJS applications. After that, I prefer explicit solutions. Redux is intuitive. All possible transitions in the state are described within the reducers. Reducers are activated by actions. Finally, actions are emitted by interactions. The beauty of reactivity.
Conclusions
I was under huge impression how easy I can write a full client application without worrying about the server-side yet. I prepared some actions dedicated to gathering data from the server, but now instead of doing request, I resolved a delayed promise with dummy data. I know all these things were possible but I never tried work in this way before.
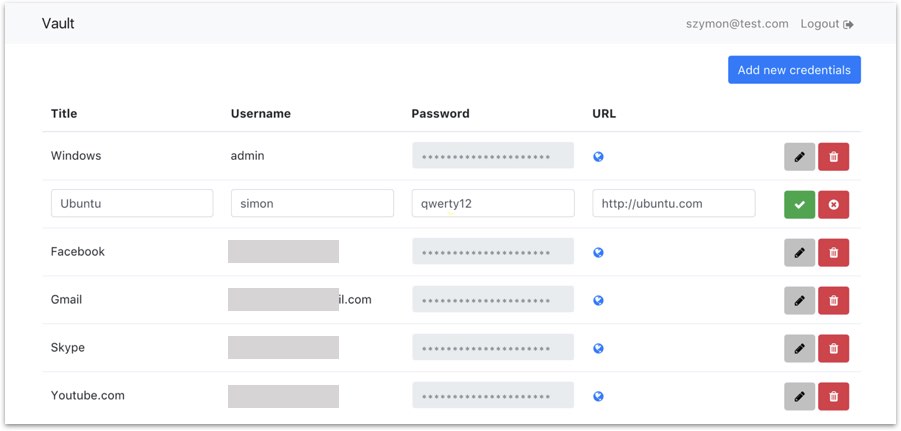
Building with React gives me a lot of fun because it’s interrelated to object-oriented programming as well as to functional programming. It depends on who handles the state management. I also say building instead of programming. I notice a majority of work is a compose bigger components from smaller ones. It may be an illusion because lots of logic is hidden within their as well as within the actions and reducers.
The one thing I miss is tests, because of limitation of time I focus mostly on building the app rather than getting to know the full process. Because of that, I know I have something to catch up in this area.
Featured photo by Braden Collum on Unsplash