Blackboxing JavaScript using Chrome DevTools
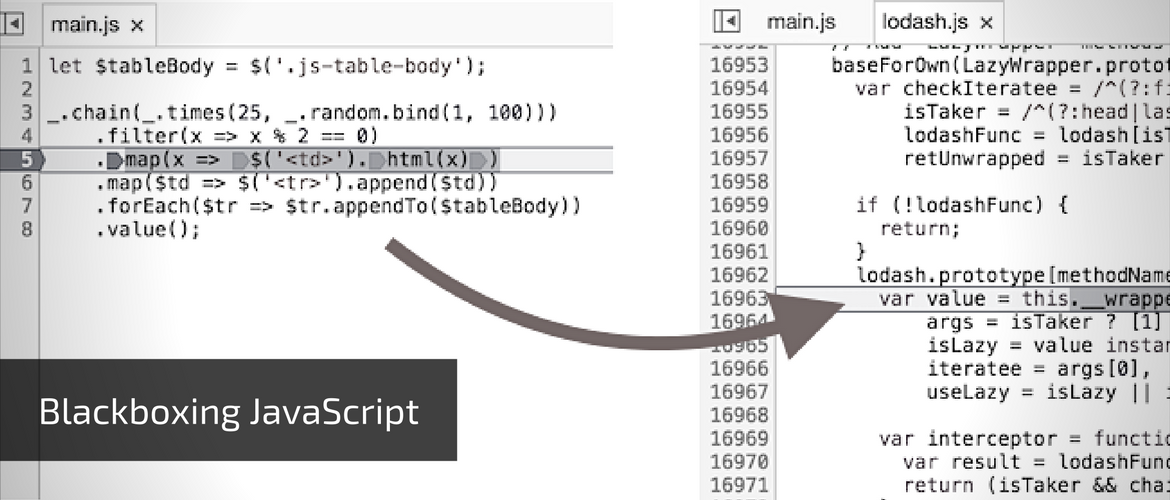
Lots of JavaScript projects use a dozen of various frameworks, libraries, and third-party scripts. More often code which you produce largely depends from vendors’ work. The good example is jQuery and Lodash libraries which are involved in our pretty code. They are designed to facilitate the work and they are the remedy for especially commonly used, repeatable pieces of code.
This kind of libraries (and frameworks) provide an another abstraction layer behind our code making the execution process more complex. It’s invisible until you’ll need to debug your code because of errors.
Problem
Let’s consider this simple snippet:
let $tableBody = $('.js-table-body');
_.chain(_.times(25, _.random.bind(1, 100)))
.filter(x => x % 2 == 0)
.map(x => $('<td>').html(x))
.map($td => $('<tr>').append($td))
.forEach($tr => $tr.appendTo($tableBody))
.value();
This part of code takes advantage of jQuery and Lodash libraries and simply put rows with the random even number into the table. Unfortunately, this logic is mixed together with third party functions. If you put the breakpoint trying to debug the flow of the chain step by step, you’ll be flipped to the library code.
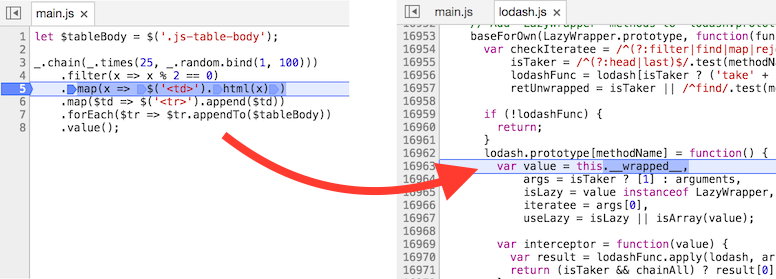
Solution
To avoid exploring the third party code during debugging you can use the feature from Chrome DevTools called Blackboxing. When you reach the file you have to omit, call the context menu on source code and use the option Blackbox Script.
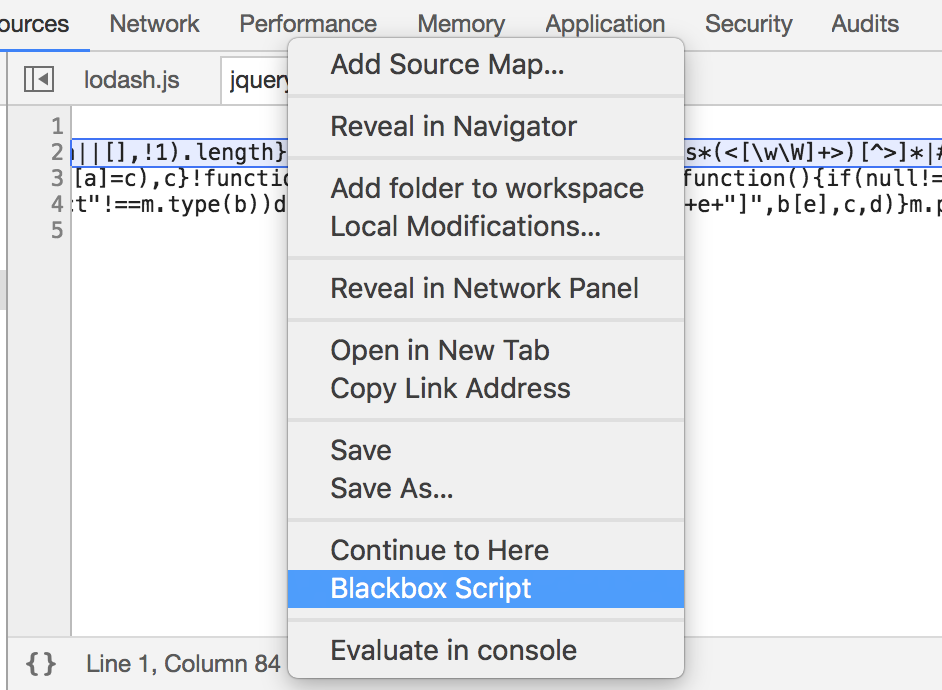
In Call Stack, you’ll have an information that some frames are hidden because of blackboxing the script. Now if you use Step into next function call the debugger will skip all unnecessary frames.
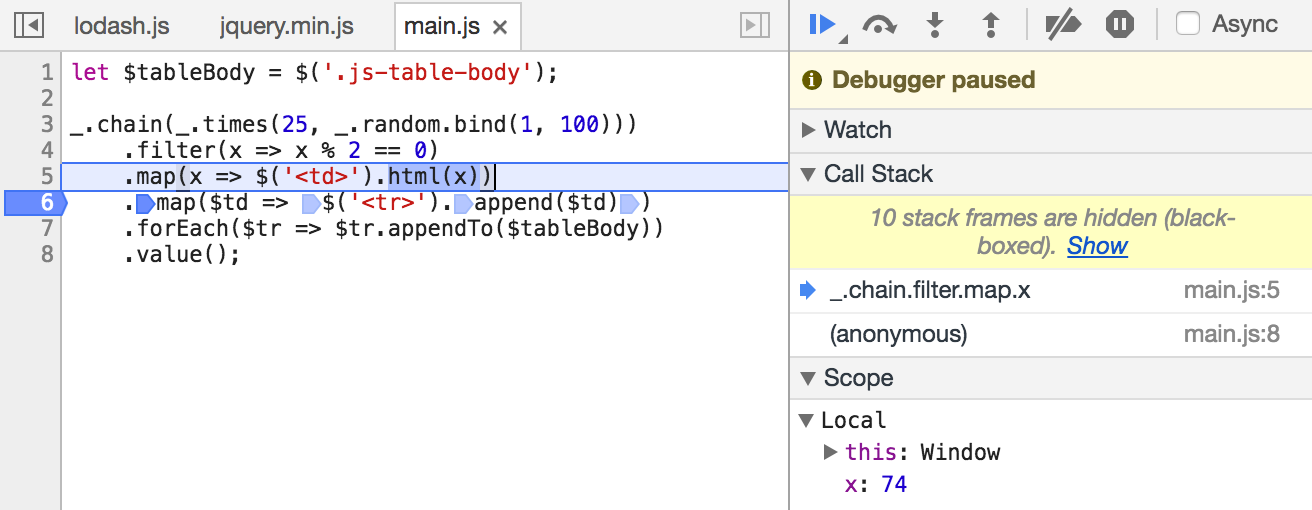
If you want to see the list of blackboxed scripts, you’ll simply go to Chrome DevTools -> Settings -> Blackboxing. You can also add the new blackboxed script by hand.
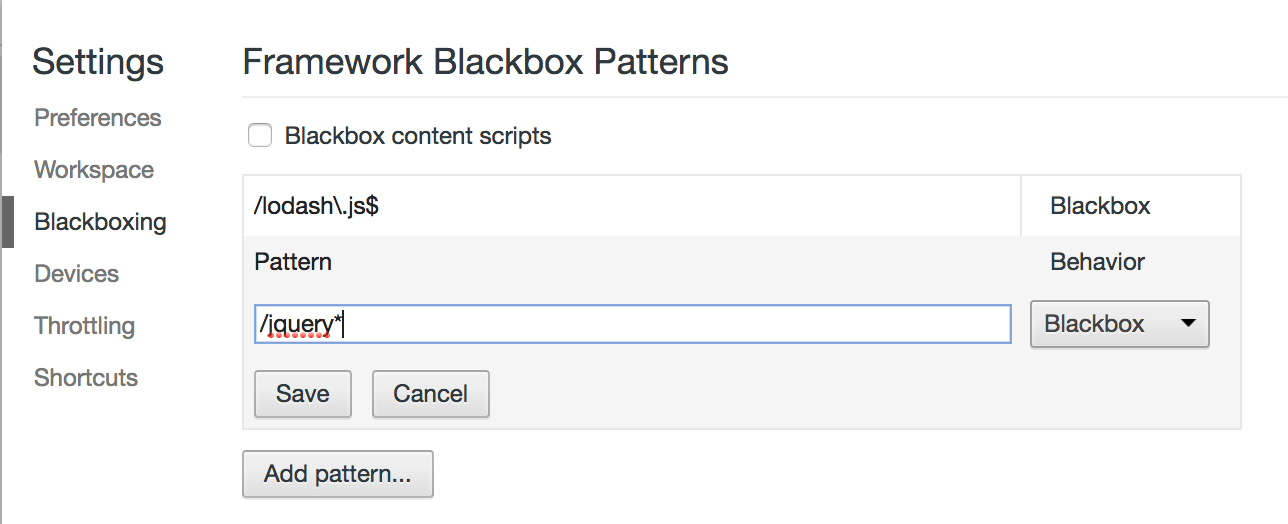
Tip: Use regular expressions in pattern to blackbox common scripts
As long as you don’t need to dig into some libraries or frameworks you can put them into the blackbox. Prepare your list and put into the pattern list. You can also omit all minified files by using this regular expression
/\.+\.min\.js$
Summary
This is one of the many features in Chrome DevTools that I haven’t used before because I haven’t known it even exists. It only shows that you should get to know better with tools which you use in your work. They are to make your work easy and fast.